Printing output on console in Go
This article addresses printing output in Go by using the print
function. As in Unix and C, Go also provide a variety of ways for printing your output on screen or console. All these functionalities can be done by using the fmt
Go package. The fmt
Go package implements I/O functions that are comparable to C’s printf
and scanf
. The syntax are copied from C but much simpler in Go. For more info, read fmt Go package.
To easiest way to get started with the fmt
Go package for printing, use the println
and printf
function:
Example using Println:
package main
import "fmt"
func main() {
fmt.Println("Go dev tips is awesome!")
}
Example using Printf:
import "fmt"
func main() {
stringValue := "Go dev tips!"
fmt.Printf("Hello %s",stringValue)
}
The print
function uses a default formats for each value in the order specified. In case any error occurred with the provided format it will throw an error. The fmt.Print()
function is identical to the fmt.Println()
, but there is two main difference:
- New line will not be added at the end of each line
- Space between arguments will only be added if neither is a string
Function signature:
fmt.Println(a ...interface{}) (n int, err error)
package main
import "fmt"
func main() {
fmt.Print("Go dev tips ","is ","awesome")
// output -> Go dev tips is awesome
}
In case all characters are non-string spaces will be added between each one automatically:
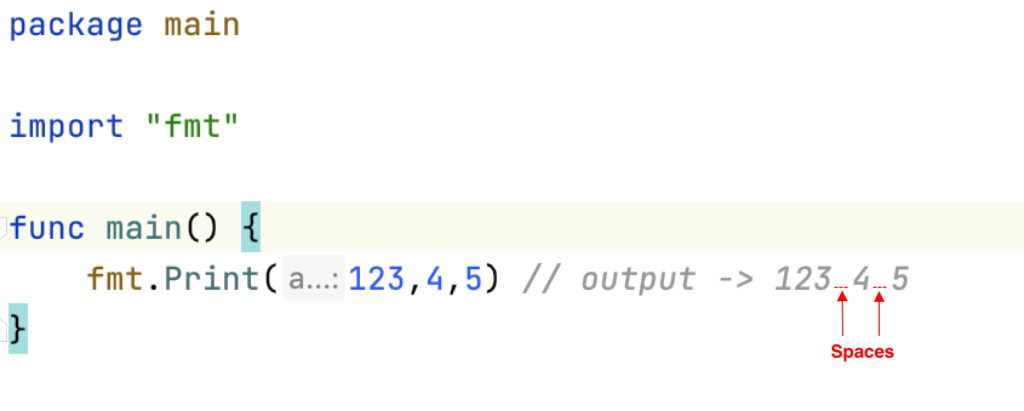
The fmt.Print()
function can also be used instead of fmt.println()
. The main different between fmt.Print()
and fmt.Println()
is that when using the fmt.Println()
function a newline character will be added each time you use it, and the fmt.Print()
function does not.
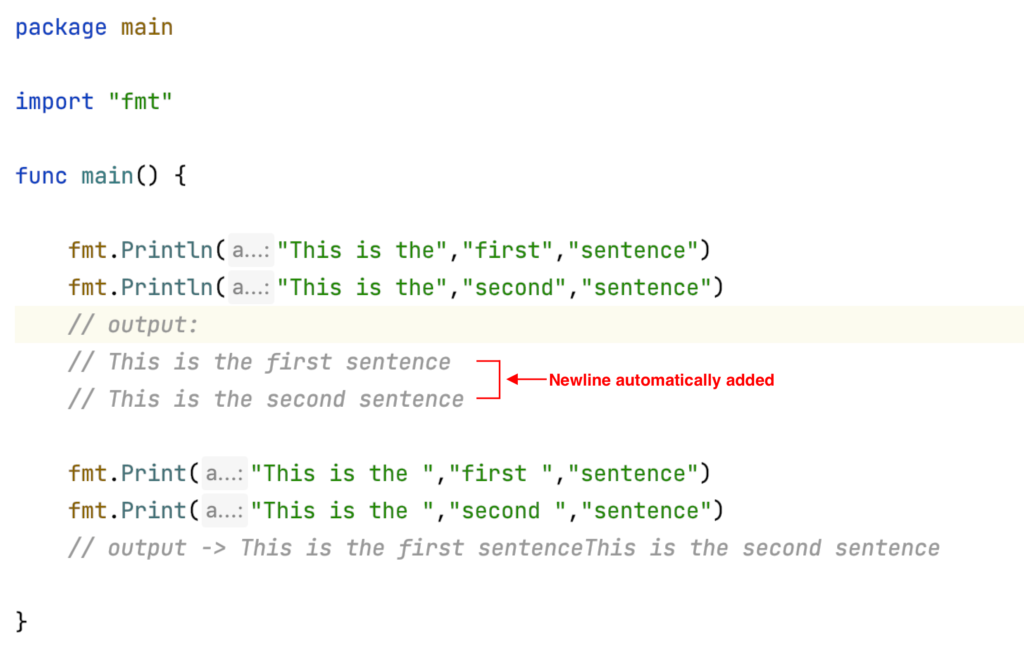
Tips:
If you only have to print a single variable and you need to choose between fmt.println()
and fmt.print()
it will only depends on whether you need the newline character or not.
Printf
The fmt.printf()
function requires a format specifier and for each one, you must add the value which must corresponds to the data type expected by the format specifier. With such function you have a much better control but on the other hand requires more codes.
package main
import "fmt"
func main() {
binaryValue := 0100
fmt.Printf("Binary format: %b",binaryValue)
// output: Binary format: 1000000
}
Format specifier
The following table illustrate the format specifier possibilities:
Format specifier | Definition |
%b | – For base 2 numeral system (Binary numeral system) – In case of floating-point and complex components: decimal less scientific notation with a power of two (e.g.: -123456 -78) |
%c | Unicode point character, see Generic Unicode in Go for some examples. |
%d | For base 10 numeral system integer (int , int8 , int16 , int32 , int64 , uint , uint8 , uint16 , uint32 , uint64 and uintptr )– Int : 32 bit signed integer– Int8 : 8-bit signed (range: -128 -> 127)– Int32 : 32-bit integer (range: -2147483648 -> 2147483647)– Int64 : 64-bit integer (range: -9223372036854775808 -> 9223372036854775807)– Uint : unsigned type 32 bits– Uint8 : unsigned 8-bit integer (range: 0 -> 255)– Uint16 : unsigned 16-bit integer (range: 0 -> 65535)– Uint32 : unsigned 32-bit integer (range: 0 -> 4294967295)– Uint64 : unsigned 64-bit integer (range: 0 -> 18446744073709551615)– Uintptr : unsigned 64-bit integer |
%o | Base8-numeral system well-known as Octal: digit 0 – 7 integers |
%O | Base8-numeral system ‘Octal’ with ‘0o’ prefix |
%q | A single-quoted escape |
%x | Base 16 numeral system lower case hexadecimal, see Printing hexadecimal in Go |
%X | Base 16 numeral system upper case hexadecimal, see Printing hexadecimal in Go |
%U | Unicode format, see Printing hexadecimal in Go |
%e | Scientific notation exponent (e.g.: -1.234456e+78) |
%E | Scientific notation exponent (e.g.: -1.234456E+78) |
%f, %F | Decimal point with no exponent (floating-point). Precision: – %f : default width (default precision)– %9f : width 9 (default precision)– %.2f : default width (precision 2)– %9.2f : width 9 (precision 2)– %9.f : width 9 (precision 0) |
%g | Same as %e but for large exponents |
%G | Same as %E but for large exponents |
%x | For floating point: – hexadecimal notation decimal power of two exponent (e.g.: 0x1.3ep+42) For slices or strings: – base 16 lower-case (two character per byte) |
%X | For floating point: – Upper-case hexadecimal notation decimal power of two exponent (e.g.: 0x1.3ep+42) For slices or strings: – base 16 upper-case (two character per byte) |
%s | Bytes of string slice |
%q | Double-quoted string escape format |
%p | First element in base 16 annotation with prefix ‘0x’. Note: %b ,%d ,%o , %x and %X also work with pointers. The value will be formatted exactly as an integer |
%t | Boolean |
%v | Value in a default format that can be used with: – %t – %d (‘%#x ‘ if printing with ‘%#v ‘)– %g – %s – %p |
\b | Backspace (U0008 ) |
\\ | Backslash (U005c ) |
\t | Horizontal tab (U0009 ) |
\n | New line (U000A ) |
\f | Form feed (U000C ) |
\r | Carriage return (U000D ) |
\v | Vertical tab (U000b ) |
Advance format specifier examples
Format specifier | Description | Example |
%+d | Show plus sign | ‘+105’ |
%<width>d | Pad with spaces (total width with padding on the left side in case the value is less than the width specified) | %5d -> __105 |
%-<width>d | Pad with spaces (total width with padding on the right side in case the value is less than the width specified) | %-5d -> 105__ |
%0<width>d | Pad with zeroes based on the width provided | %05d -> 00105 |
%#x | Base 16 with leading 0x | 105 -> 0x69 |
%#U | Unicode with character | 105 -> U+0069 ‘i’ |
%<width>s | Total width with padding on the left side in case the value is less than the width specified | %8s -> “___value” |
%-<width>s | Total width with padding on the right side in case the value is less than the width specified | %-8s -> “value___” |
Error handling printf
Invalid argument
When using the printf
with format specifier it throws an error when an invalid argument is provided. An example of such scenario is providing an integer where a string is expected.
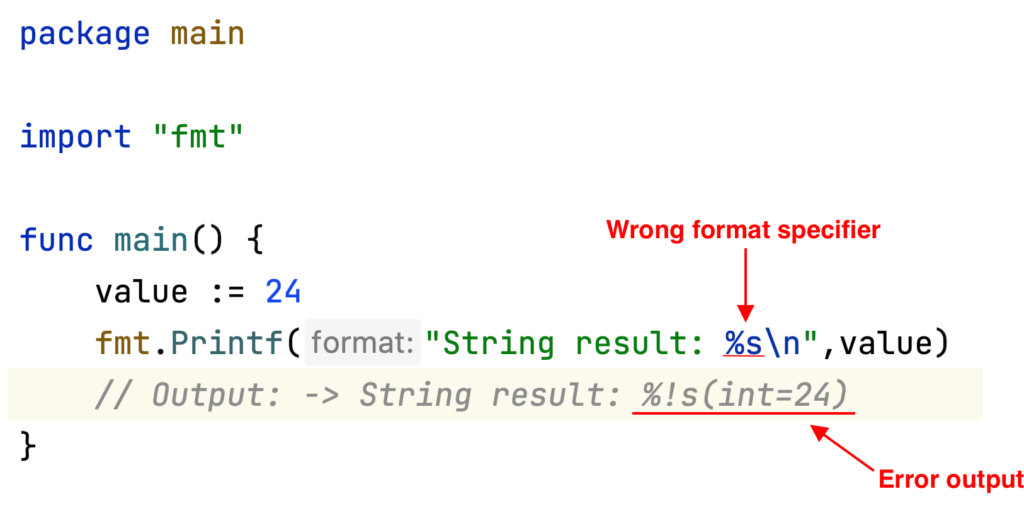
All errors begin with string “%!” and sometimes by a single character and end with parenthesized description. In this case it was expecting a string but encounter an int of the value 24.
Missing arguments error
When a format specifier is provided with no argument, it will throw a missing error output like in the illustration below.
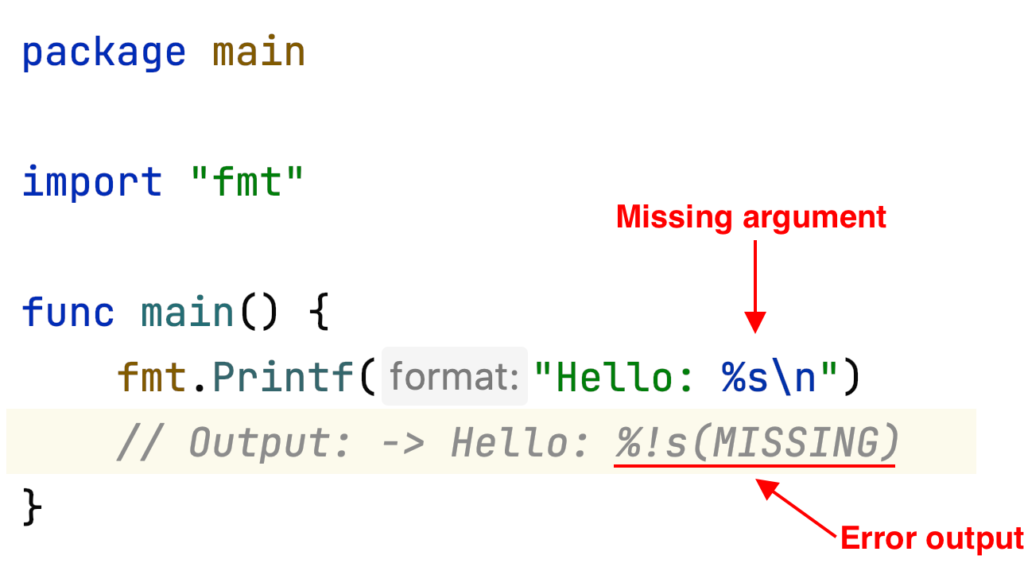
Too many arguments error
This error occurs when more argument is provided than format specifier(s).
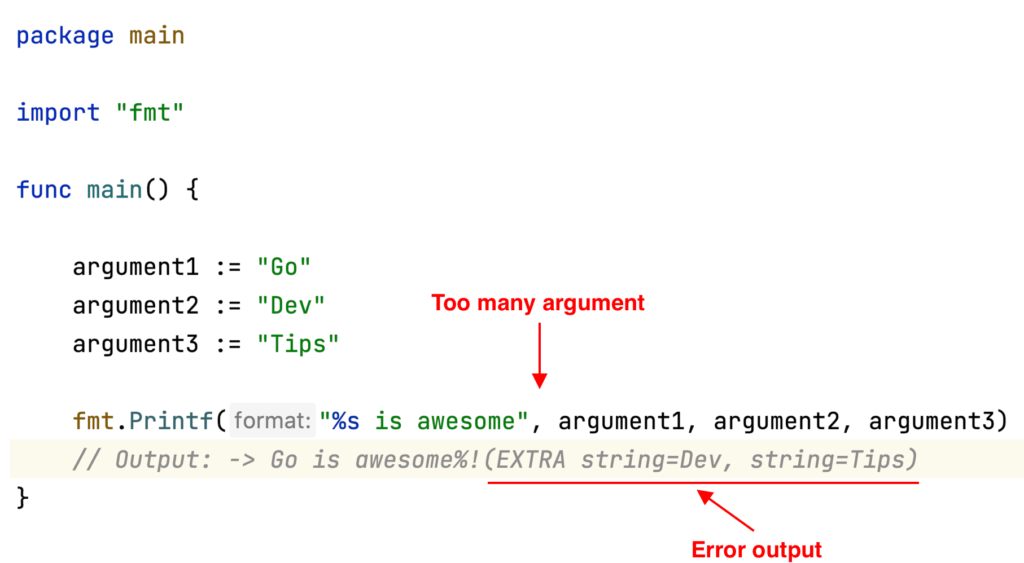
Non-int width error
With a format specifier you can also define a custom width for the corresponding string by using the ‘%*s
’. A custom width expects an Int value and if you define a non-int value it will throw an BADWIDTH error.
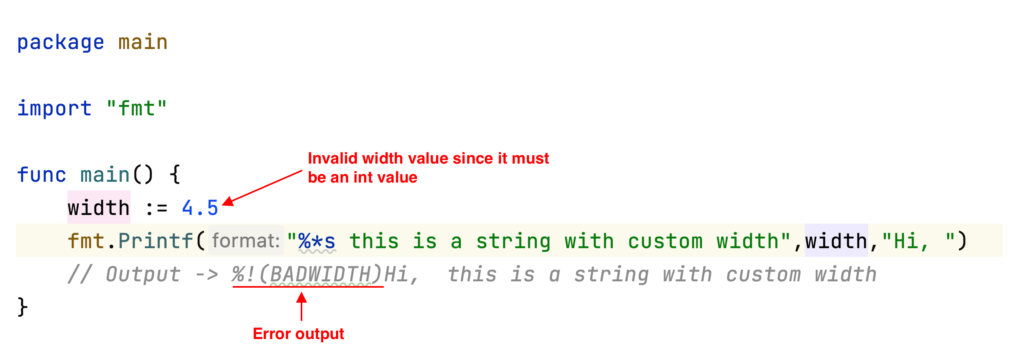
Non-int precision error
With a format specifier you can also define a custom precision for the corresponding string by using the ‘%.*s
’. A custom precision expects an Int value and if you define a non-int value it will throw an BADPREC (bad precision) error.

Invalid index error
Defining argument index is also allowed when using printf
function by using the ‘%*[index]d
’. Such format specifier defines an integer array and the index on which the value must be retrieved.
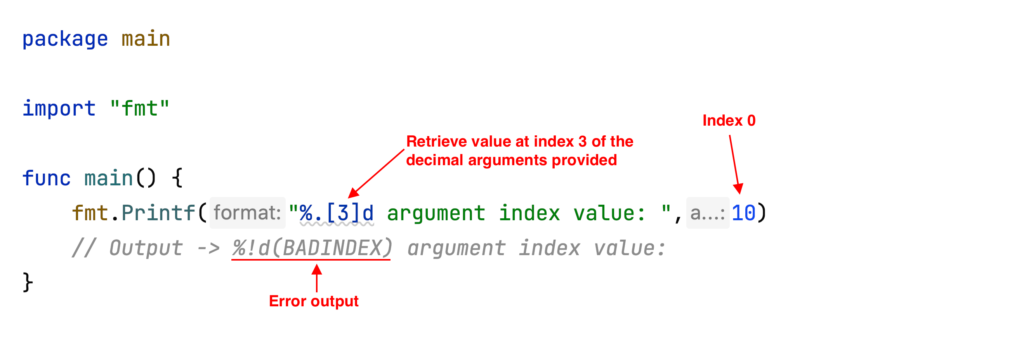
Panic error
Some of the function can throw a panic when a fatal error occurs, such example is when the value provided is equal to nil. In case of the string value the following error message will be displayed: “<nil>”.
Println
The println
function print data to console. When using this function, a new line will be added at the end of every line.
fmt.Println(...interface{}) (n int, err error)
Based on the signature of the function it contains interface which means it takes a number of arguments of any type.
package main
import "fmt"
func main() {
fmt.Println("Go dev tips is awesome!")
}
‘Vet command’ for finding suspicious codes
The vet command examines Go source code and reports suspicious constructs. With all the error examples provided in the above sections, this command is able to capture error that maybe is not caught by the compiler.
This command can be used by the following code command:
go vet <project file>
In this following example we are going to check the print_f.go
file for any error. The error results indicates that at line 13 there is a Sprintf
function with two argument instead of one:

Follow us:
Pingback:котел цена